27.
非同期処理1
Web APIとJSON
Web APIとは
Web API (Web Application Programming Interface) は、Web上で利用可能なプログラム間のインターフェースを指します。Web APIを利用することで、さまざまなアプリケーション間(Webサーバ、PC、モバイル)のデータ連携が可能になります。
HTTP、HTTPSプロトコル
Web APIは通常、HTTPやHTTPSプロトコルを使ったインターネット通信のことで、Webサーバとクライアント間でのリクエスト&レスポンスで動作します。
REST API
REST API(Representational State Transfer API)は最も一般的なWeb API設計で、HTTPリクエスト(GET、POST、PUT、DELETEなど)でサーバにアクセスし、URLパスとクエリパラメータでデータ取得・送信します。
ユーザーID 123 の情報を取得
GET https://api.example.com/users/123
GraphQL API
GraphQL APIは比較的新しいAPI通信で、クライアントが必要なデータ条件を具体的にしてリクエストします。
query {
user(id: "123") {
name
email
}
}
SOAP API
SOAP API (Simple Object Access Protocol)は、XMLを利用した重厚なAPIプロトコルで、特に古いシステムや企業向けのシステムで使用されています。
データフォーマット
Web API 通信で利用される主なデータフォーマットには、以下のような形式があります。
フォーマット | 軽量性 | 可読性 | 速度 | 用途 |
---|---|---|---|---|
JSON | 高い | 高い | 高速 | REST, GraphQL, モバイル/Web通信 |
XML | 低い | 中程度 | 中程度 | SOAP, エンタープライズ通信 |
YAML | 中程度 | 高い | 中程度 | 設定ファイル, インフラ構成管理 |
CSV | 高い | 高い | 高速 | データベース, 表形式のデータ |
Protobuf | 高い | 低い | 非常に高速 | gRPC, 高パフォーマンス通信 |
Avro | 高い | 低い | 高速 | ビッグデータ, ストリーム処理 |
MessagePack | 高い | 中程度 | 非常に高速 | IoT, モバイル通信 |
XMLとは
XML(eXtensible Markup Language) は、構造化データを記述するためのマークアップ言語で、データ保存や転送、アプリ開発の設定ファイルなどに利用されています。特定のアプリケーションやプラットフォームに依存しない形でデータをやり取りすることを目的としています。
<user>
<name>John</name>
<age>30</age>
<city>New York</city>
</user>
<user name="John" age="30" city="New York" />
JSONとは
JSON(JavaScript Object Notation)は、JavaScriptの標準的なテキストベースのデータ形式です。人間が読み書きしやすく、様々なプログラムやプログラミング言語で利用されています。
アプリやAPIで利用
JSONは、Webアプリケーション、API、スマホなど、さまざまなアプリケーションで広く使用されています。
JSON
{"name":"John","email":"[email protected]","birthday":"1980/10/04","city":"New York"}
XMLとの比較
特徴 | XML | JSON | GraphQL |
---|---|---|---|
用途 | データ交換、文書構造の記述 | APIデータの軽量データの取得 | APIデータの効率的な取得 |
可読性 | 高い | 高い | 高い |
データサイズ | 多い | 少ない | 少ない |
パーサ | 専用パーサ | 多くの言語でネイティブサポート | 専用ライブラリ |
データ表現方法 | 階層的なタグ構造 | オブジェクトや配列 | クエリ言語 |
データ取得 | XPathやDOM操作が必要 | アクセスが比較的簡単 | クエリ言語て必要なデータだけ取得 |
主な使用場面 | ドキュメント保存、設定ファイル、SOAPメッセージ | REST API、データ交換、APIデータ通信 | APIデータ通信 |
JSONのメリット
人間に分かりやすい
JSONは、構文がシンプルで直感的なため、データ構造を簡単に理解できます。
プログラム処理しやすい
JSONはキーと値のペアでデータを構造化なので、プログラムによる解析と処理が容易です。
{
"name": "John",
"email": "[email protected]",
"birthday": "1980/10/04",
"city": "New York"
}
基本的なデータ型をサポートしています。
- オブジェクト(連想配列)
- 配列
- 文字列
- 数値
- 真偽値
- null
データの軽量化
JSONはXMLなどと比較してフォーマットがシンプルです。データ量が少ないため通信速度の向上やストレージ容量の節約が可能です。
言語やプラットフォームに依存しない
JSONはJavaScript以外のほとんどのプログラミング言語(Python, Java, C#, PHPなど)でサポートされています。 JSONを利用することで、異なる言語間でデータを簡単にやり取りできます。
JSONデータの操作
ファイル構成
app1.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>User Info</title>
<script src="https://cdn.tailwindcss.com"></script>
</head>
<body class="container mx-auto flex flex-col items-center">
<div class="w-2/3 mt-5 p-6 rounded shadow">
<h2 class="text-2xl text-center p-3">ユーザデータ(JSオブジェクト)</h2>
<div id="person-container" class="space-y-4">
<div class="flex">
<label for="user-name" class="w-1/4 font-bold">Name:</label>
<input id="user-name" type="text" class="flex-1 p-2 border border-gray-300 rounded">
</div>
<div class="flex">
<label for="user-email" class="w-1/4 font-bold">Email:</label>
<input id="user-email" type="text" class="flex-1 p-2 border border-gray-300 rounded">
</div>
<div class="flex">
<label for="user-city" class="w-1/4 font-bold">City:</label>
<input id="user-city" type="text" class="flex-1 p-2 border border-gray-300 rounded">
</div>
<div class="text-center">
<button
id="update-button"
onclick="update()"
class="mt-3 px-4 py-2 bg-blue-500 text-white rounded shadow hover:bg-blue-600">
更新
</button>
</div>
</div>
</div>
<div class="w-2/3 mt-5 p-6 rounded shadow">
<h2 class="text-2xl">JSON</h2>
<div id="json-user" class="p-2 w-full border border-gray-300 rounded">
</div>
</div>
<script id="data" type="application/json">
{
"name": "John",
"email": "[email protected]",
"city": "New York"
}
</script>
<script defer src="js/app1.js"></script>
</body>
</html>
JSON.parse()
JSでJSONデータを処理するには、JSONオブジェクトを利用します。JSON.parse() は、JSONデータをJavaScriptオブジェクトに変換するメソッドです。
JSON.parse(jsonString);
JSONデータ読み込み
HTMLに記述されているJSONデータを読み込みます。
app1.html
<script id="data" type="application/json">
{
"name": "John",
"email": "[email protected]",
"city": "New York"
}
</script>
js/app1.js
var jsonString = document.getElementById('data').textContent;
- 実際のJSONデータは、Web APIサーバから取得します。
オブジェクトに変換
JSON.parse() でJSONデータをオブジェクトに変換します。
js/app.js
var user = JSON.parse(jsonString);
データ表示
displayUser() を定義してユーザ情報を表示します。
js/app.js
var user = JSON.parse(jsonString);
displayUser(user);
function displayUser(user) {
document.getElementById('user-name').value = user.name || '';
document.getElementById('user-email').value = user.email || '';
document.getElementById('user-city').value = user.city || '';
}
ユーザオブジェクトが表示されました。
ブラウザ
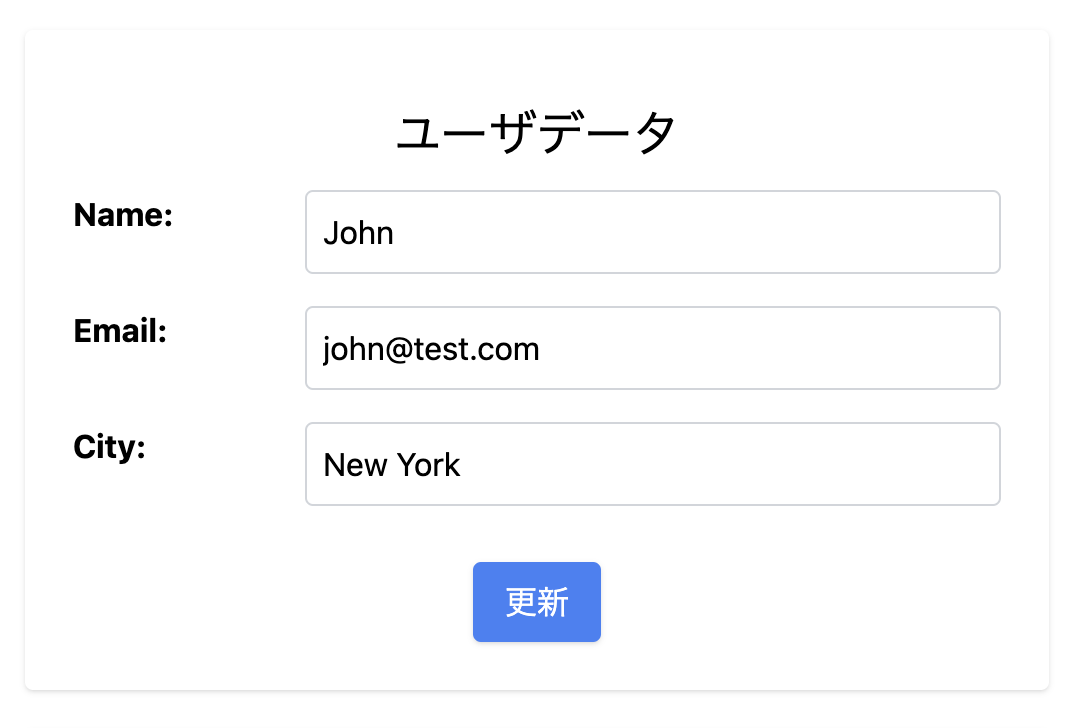
JSON.stringify()
JSON.stringify() は、オブジェクトをJSONに変換するメソッドです。
JSON.stringify(value);
データ更新
update() を定義してuserオブジェクトをJSONに変換し、ブラウザにテキスト表示します。
js/app1.js
function update() {
user.name = document.getElementById('user-name').value;
user.email = document.getElementById('user-email').value;
user.city = document.getElementById('user-city').value;
// オブジェクト -> JSON
document.getElementById('json-user').textContent = JSON.stringify(user);
}
ブラウザで情報を入力して【更新】すると、JSONがテキスト表示されました。
ブラウザ
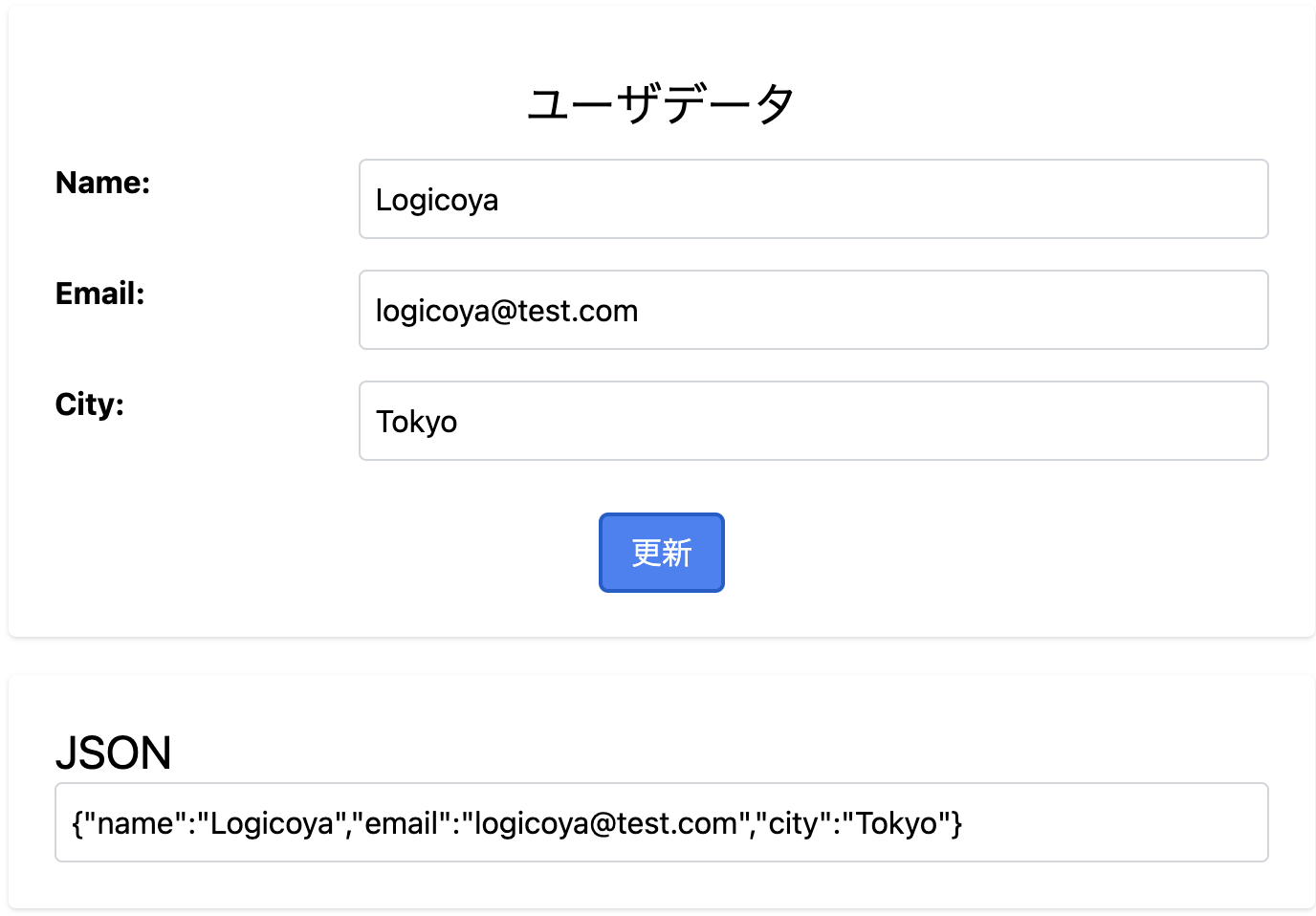
- 実際には、このJSONデータをWeb APIサーバに送信します。
非同期処理
非同期処理とは
JavaScriptの非同期処理は、時間のかかる処理(例: データ取得やファイル操作)を実行中に、アプリケーション全体の動作を止めることなく、他のタスクを同時に実行できる仕組みを指します。非同期処理を活用することで、UIがフリーズせず、スムーズなユーザー操作ができます。
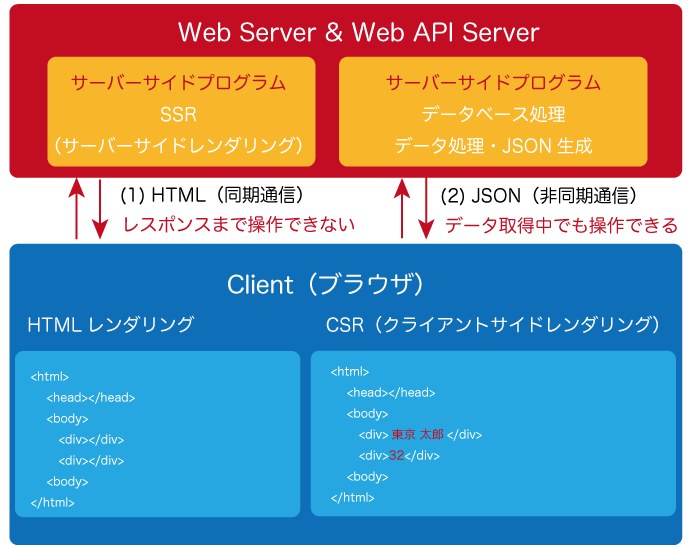
非同期処理のシチュエーション
非同期処理はネットワーク接続やファイル読み込みするときに利用します。例えば、Webページでバックエンドサーバーとデータ取得中でも、ユーザが別の操作ができます。
非同期処理の種類
JavaScriptの非同期処理はいろいろな構文で処理できます。
- コールバック関数(callback)
- XMLHttpRequest(XHR)
- プロミス(Promise)
- 非同期/待機キーワード(async/await)
XMLHttpRequest
XMLHttpRequestとは
XMLHttpRequest(XHR)は、ウェブページからサーバーにリクエストし、非同期でデータ取得するAPIです。
XHRオブジェクト
XMLHttpRequestは、インスタンスを生成して利用します。
const xhr = new XMLHttpRequest();
open()
open() は、HTTPリクエストを設定および初期化するメソッドです。 HTTPメソッド(GET、POSTなど)、URL、非同期・同期のの設定を指定します。
xhr.open(HTTPメソッド, URL, async[, user[, password]]]);
send()
open() 実行後、send() でサーバにリクエスト送信します。
xhr.send();
onload
リクエストが完了すると、onloadコールバック関数が実行されます。
xhr.onload = function () { }
XMLHttpRequestで処理
「pertons.json」にWebサーバ経由リクエストし、JSONデータを非同期処理で取得します。
ファイル構成
├── data
│ └── persons.json
├── js
│ └── xhr.js
└── xhr.html
data/persons.json
[
{ "name": "Alice", "age": 25, "city": "LA" },
{ "name": "Bob", "age": 28, "city": "CA" },
{ "name": "John", "age": 30, "city": "NY" }
]
API URL生成
現在のURLから、APIデータのURLを生成します。
function getApiURL() {
const currentURL = location.href
const fileName = currentURL.substring(currentURL.lastIndexOf('/') + 1);
const baseURL = currentURL.replace(fileName, '');
return baseURL + 'data/persons.json';
}
const API_URL = getApiURL();
console.log(API_URL)
コンソール
http://localhost/xxxx/data/persons.json
JSONデータリクエスト
XMLHttpRequest で、外部JSONデータを取得します。
xhr.js
const xhr = new XMLHttpRequest();
xhr.open('GET', API_URL, true);
xhr.onload = function () {
if (xhr.status === 200) {
const json = xhr.responseText;
console.log(json);
} else {
console.error('リクエストが失敗しました。');
}
};
xhr.send();
JSONデータが取得できました。
コンソール
[
{ "name": "Alice", "age": 25, "city": "LA" },
{ "name": "Bob", "age": 28, "city": "CA" },
{ "name": "John", "age": 30, "city": "NY" }
]
データ処理
JSONデータをオブジェクトに変換(パース)し、データを繰り返し表示します。
const xhr = new XMLHttpRequest();
xhr.open('GET', API_URL, true);
xhr.onload = function () {
if (xhr.status === 200) {
const json = xhr.responseText;
console.log(json);
//オブジェクトに変換
const persons = JSON.parse(json)
for (const person of persons) {
console.log(person.name)
}
} else {
console.error('リクエストが失敗しました。');
}
};
xhr.send();
コンソール
Alice
Bob
John