テンプレートエンジン
テンプレートエンジンとは
テンプレートエンジンは、HTMLにJavaプログラムを埋め込んでコーディングできる機能で、MVCのビューとして利用します。
テンプレートエンジンの種類
Springのテンプレートエンジンは、Thymeleafが推奨となっています。
言語 | テンプレートエンジン・マークアップ |
---|---|
Java | Thymeleaf、JSP |
PHP | Blade、Smarty、Twig |
JavaScript | EJS、Pug |
Ruby | Haml |
Python | jinja、Djangoテンプレートエンジン |
Thymeleafとは
Thymeleafは、Javaで人気の高いテンプレートエンジンの1つです。
ライブラリ依存追加
Mavenを使用している場合、「pom.xml」にライブラリ依存の設定が必要です。
- 今回は、Springプロジェクト作成時に選択インストール済み
pom.xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
templatesフォルダ
ThymeleafではHTMLテンプレートファイルをsrc/main/resources/templates/ に配置します。
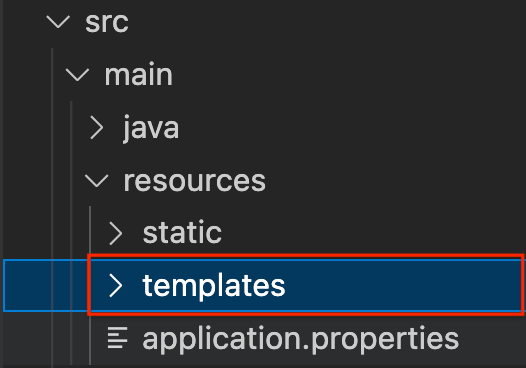
- テンプレートフォルダのパスは、application.properties で設定変更可能
テンプレートファイルのパス
ビューを表示するには、return でテンプレートファイルのパスを指定します。テンプレートファイルのパスは、src/resouces/templates/ と拡張子を省略した文字列で指定します。
return ファイルパス;
以下は「src/resouces/templates/home/sample.html」をレンダリングする場合です。
return "home/sample";
thアトリビュート
Thymeleafでは、th: で始まる専用タグを利用することで、モデルやコントローラなどで処理したデータをHTMLでプログラミングすることができます。
<table>
<thead>
<tr>
<th th:text="#{msgs.headers.name}">Name</th>
<th th:text="#{msgs.headers.price}">Price</th>
</tr>
</thead>
<tbody>
<tr th:each="prod: ${allProducts}">
<td th:text="${prod.name}">Oranges</td>
<td th:text="${#numbers.formatDecimal(prod.price, 1, 2)}">0.99</td>
</tr>
</tbody>
</table>
ビュー作成
トップページ作成
HTMLファイル作成
templates/ に homeフォルダを作成します。
templates/home/ に、sample.htmlを作成します。
templates/home/sample.html
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My News</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" th:href="@{/css/style.css}">
</head>
<body>
<main class="container">
<div>
<h2>最新ニュース</h2>
<div>
<h3>ニュースタイトル1</h3>
<p>
<span>2023/07/01 13:15</span>
</p>
</div>
</div>
</main>
</body>
</html>
コントローラ修正
@RequestBody削除
HTMLテンプレートをレスポンスできるように、HomeController->index() の定義で、@RequestBody は不要なので削除です。
controllers/HomeController.java
@Controller
public class HomeController {
@GetMapping("/")
//@RequestBody <- 削除
public String index() {
return "This is Top Page";
}
}
home/sample.html の表示
index() で「/home/sample」を返します。
controllers/HomeController.java
@GetMapping("/")
public String index() {
return "home/sample";
}
「templates/home/sample.html」を自動で読み込みます。
http://localhost:8080/ にアクセスして、HTMLが表示されるか確認してみましょう。
結果
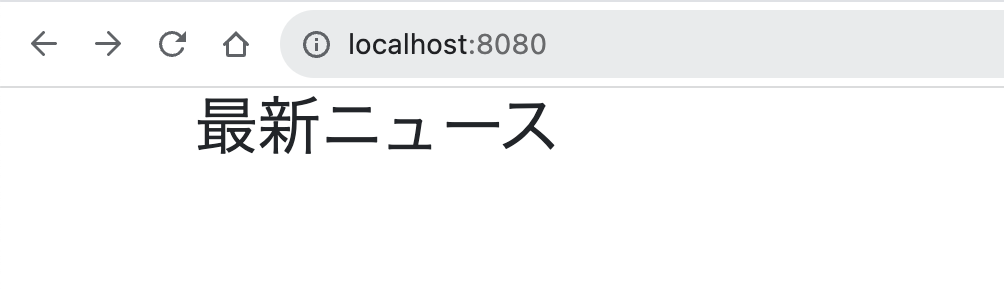
管理者ページ
ファイル構成
controllers/
├── admin/
│ ├── HomeController.java
ルーティング
URLパターン | コントローラー | アクションメソッド | リクエストメソッド | ビュー |
---|---|---|---|---|
/admin/ | admin.HomeController | index | GET | admin/index.html |
コントローラー作成
「controllers/」に「admin」フォルダを作成し、その中に「HomeController」を作成します。
コントローラ名の指定
「HomeController」というコントローラー名はすでに利用されているため、@Controllerで「AdminHomeController」と指定します。
controllers/admin/HomeController.java
package com.example.demo.controllers.admin;
import org.springframework.stereotype.Controller;
//コントローラー名の指定
@Controller("AdminHomeController")
public class HomeController {
}
@RequestMappingの共通化
@RequestMappingをコントローラークラス全体に定義すると、共通のURLパス(パスプレフィックス)で処理できます。「AdminHomeController」のアクションメソッドはURLパス「/admin/xxxx」でアクセスできるように共通化します。
controllers/admin/HomeController.java
@Controller("AdminHomeController")
//URLパスの共通化
@RequestMapping("/admin/")
public class HomeController {
}
アクションメソッド追加
アクションメソッドindex() を追加します。
@Controller("AdminHomeController")
@RequestMapping("/admin/")
public class HomeController {
@GetMapping(value = "")
public String index() {
return "admin/index";
}
}
ビュー作成
adminフォルダ作成
「templates/」に「admin」フォルダを作成します。
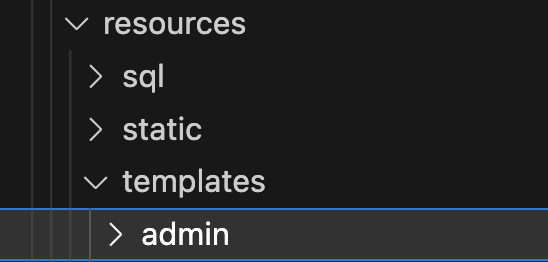
トップページテンプレート作成
「templates/admin/」にテンプレートファイル「index.html」を作成します。
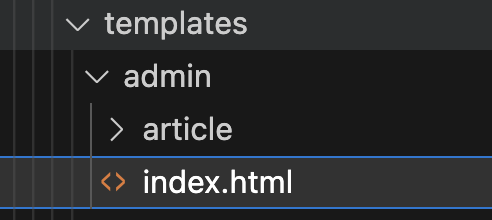
templates/admin/index.html
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My News</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" href="/css/style.css">
</head>
<body>
<main class="container">
<div class="row">
<div class="col-12">
<div>
<h2>Admin Top</h2>
</div>
</div>
</div>
</main>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
http://localhost:8080/admin/ にアクセスしてビューが表示されるか確認しましょう。
結果
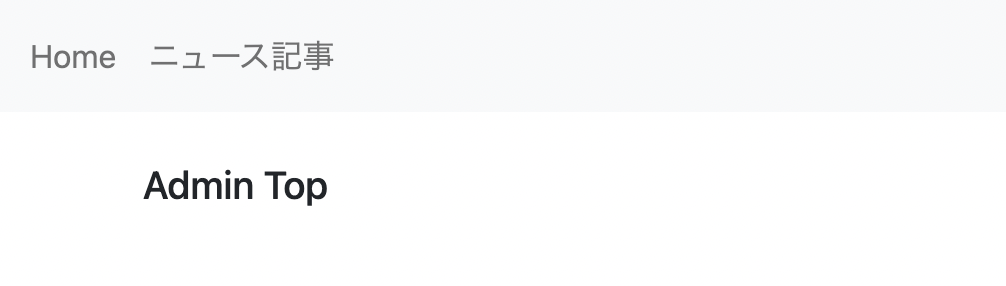